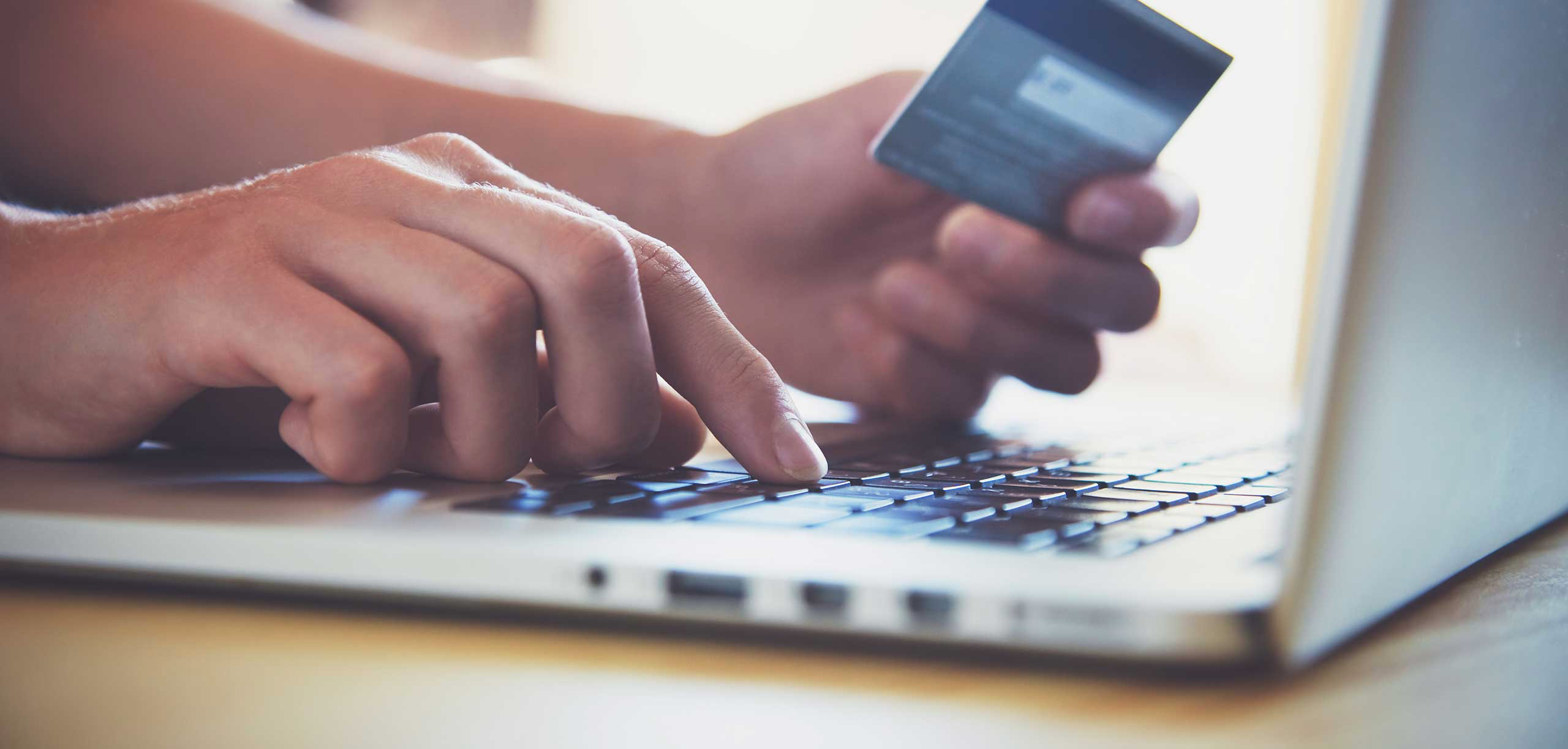
When you’re about to build a payment flow into your app, it’s important to consider payments from three different perspectives:
- How to make buying as smooth and secure as possible for your customers
- How to create a fit-for-purpose checkout process that helps you increase mobile conversions
- Whether the chosen payment partner has APIs that allow the developers to build payments easily
With those three objectives in mind, we’ll walk you through the four steps that make frictionless app payments possible.
Step 1: Make it easy for your customers to store their card details
When the Payment Highway API is called to add a card for storage a lot of things happen behind the scenes to make the payment process fast, and secure beyond PCI standards.
When a customer opens the app for the first time, they’re presented with a view to enter their card details. This happens securely on Payment Highway technical infrastructure so that no eavesdropping is possible. While entering the card number, or primary account number as the lingo goes, it’s grouped correctly and checked for correctness by the Luhn algorithm.
By validating the input right there and then, we make sure that the user doesn’t have to reach the bottom of the screen to find out if something’s wrong. This might seem like a small thing, but perform a user test on this and you’ll find it very important.
As a developer, you may use our web form or native iOS and Android SDKs. First, you open up the payment form in web or native mobile view. For example in web:
//Require the Payment Highway library var paymentHighway = require('paymenthighway'); var method = 'POST'; var testKey = 'testKey'; var testSecret = 'testSecret'; var account = 'test'; var merchant = 'test_merchantId'; var serviceUrl = 'https://v1-hub-staging.sph-test-solinor.com'; var formBuilder = new paymentHighway.FormBuilder( method, testKey, testSecret, account, merchant, serviceUrl ); // Redirection URLs for the customer's browser var successUrl = 'https://example.com/success'; var failureUrl = 'https://example.com/failure'; var cancelUrl = 'https://example.com/cancel'; var language = 'EN'; // Generate Add Card form parameters using the provided formBuilder var formContainer = formBuilder.generateAddCardParameters( successUrl, failureUrl, cancelUrl, language ); /* * Build the form for POST-request: * * Or create GET-url: * ?name=value&... */ formContainer.nameValuePairs.forEach(function(field) { var name = field.first; var value = field.second; }); // Now "formContainer" has all you need to make your html form Post to Payment Highway. // Check out below what it contains. formContainer;
Using the formContainer you will create an html form, which will lead the user’s browser to display the card payment view hosted by Payment Highway.
Step 2: Hold tight as we verify the card details with the acquirer
Next, Payment Highway connects to the acquiring institutions relevant to the transaction — depending on the card brand, merchant agreement, or processing rules set for the merchant.
Then, the card data is sent over to the acquirer so that they can check the validity of the card. After going through the card brand networks all the way to the card issuing bank, a response is sent back through the Payment Highway API, informing the backend of your app whether or not the card is valid.
Typically this takes just a couple hundred milliseconds. You’ll get the response synchronously and are good to continue with the next step.
Step 3: Securely store your customer's card details with a token
Third, Payment Highway stores the full card details in its secure storage infrastructure. In exchange for the card details, it issues a card token to your app’s backend, which allows you to charge the card when the customer wants to buy something. The backend of your app gets the card token via a simple call to tokenization.
When the user returns from the web form and gets redirected back to you return URL, your app’s backend will have a tokenization ID along with the request to be used in the next step.
//Require the Payment Highway library var paymentHighway = require('paymenthighway'); //Initialize with test merchant credentials var paymentAPI = new paymentHighway.PaymentAPI( "https://v1-hub-staging.sph-test-solinor.com", 'testKey', 'testSecret', 'test', 'test_merchantId' ); //Get a card token for a test card tokenization ID await paymentAPI.tokenization("f34c7ac0-ea5b-454b-8a7a-d08c05132653");
Now your app is ready to accept payments whenever the customer taps on it, or when a certain action takes place, e.g. when a customer starts to fill up their car at a Neste gas station.
The backend of your app only has access to the card token. The token is key for charging the customer’s card while keeping the actual card details safe. It’s no secret that the token is just a random number, more precisely a version 4 UUID. Give it to your grandma, and she can’t do anything with it. Give it to your fiercest competitor and they won’t be able to do anything with it. Simple and secure by design.
Step 4: Pay with the card token
Using the token from step 3 you are now able to charge the customer’s card. This involves two simple API calls.
You will first initialize a payment transaction, then debit the payment card behind the token:
//Require the Payment Highway library var paymentHighway = require('paymenthighway'); //Initialize with test merchant credentials var paymentAPI = new paymentHighway.PaymentAPI( "https://v1-hub-staging.sph-test-solinor.com", 'testKey', 'testSecret', 'test', 'test_merchantId' ); // Debit with the Token var token = new paymentHighway.Token('7cfb9e94-9753-44a2-9081-4d07c5a9fa36'); var amount = 1990; var currency = 'EUR'; var request = new paymentHighway.TransactionRequest(token, amount, currency); await paymentAPI.initTransaction() .then(function (init) { return paymentAPI.debitTransaction(init.id, request); }) .then(function(debit) { return debit.result; });
If you’re looking to build a similar payment flow into your app or service, check out our Sandbox and get started today.